![[C#] 2일차 (변수 선언 및 자료형, Console.WriteLine메소드)](https://img1.daumcdn.net/thumb/R750x0/?scode=mtistory2&fname=https%3A%2F%2Fblog.kakaocdn.net%2Fdn%2FeMZSY4%2FbtqTLwSsjAc%2FtpX1WMskEwUOA4VXmbqJGk%2Fimg.png)
[C#] 2일차 (변수 선언 및 자료형, Console.WriteLine메소드)C#/C#200제2021. 1. 17. 14:24
Table of Contents
4. 변수 선언 및 자료형
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace A004_Variable
{
class Program
{
static void Main(string[] args)
{
Console.Write("이름을 입력하세요 : ");
string name = Console.ReadLine();
Console.Write("나이를 입력하세요 : ");
int age = int.Parse(Console.ReadLine());
Console.Write("키를 입력하세요 : ");
float height = float.Parse(Console.ReadLine());
Console.Write("안녕하세요, ");
Console.Write(name);
Console.WriteLine("님 !");
Console.Write("나이는 ");
Console.Write(age);
Console.Write("세, 키는");
Console.Write(height);
Console.WriteLine("이군요 !");
}
}
}
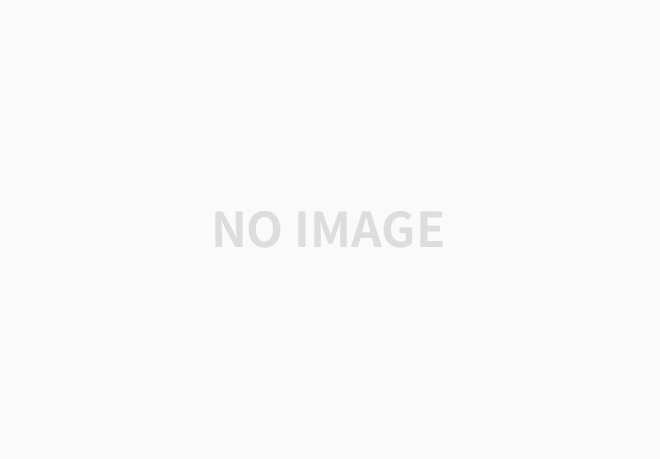
자료형중에 var형이 있다.
var형은 암시적 형식으로 지역변수로만 사용이 가능하다.
직접 선언 한것 처럼 강력한 형식이지만 컴파일러가 형식을 결정한다.
var i = 10
int 1 = 10
같게 처리함
5. 문자와 문자열
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace A005_string
{
class Program
{
static void Main(string[] args)
{
string a = "hello";
string b = "h";
b = b + "ello";
Console.WriteLine(a == b);
Console.WriteLine("b = " + b);
int x = 10;
string c = b + '!' + " " + x;
Console.WriteLine("c = " + c);
}
}
}
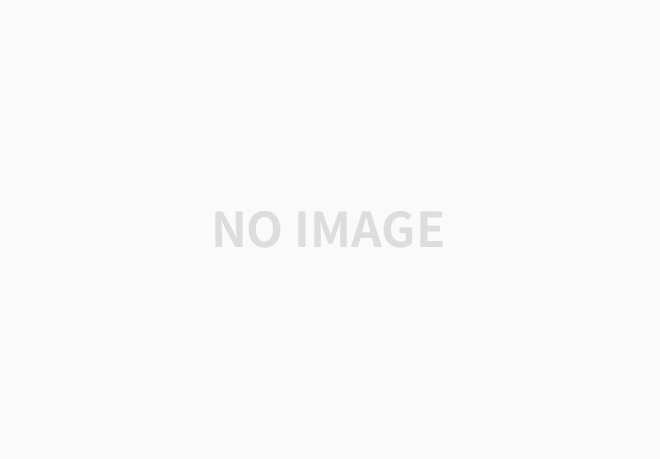
추가내용
string str = "hello";
char x = str[2]; // x = 's';
string b = @"C:\Docs\Source\a.txt"; // "C:\Docs\Source\a.txt"와 동일
Console.Write(@"abc\nabc"); // "abc\nabc"를 출력
6. 대입연산자와 대입문
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace A006_Assignment
{
class Program
{
static void Main(string[] args)
{
int i;
double x;
i = 5;
x = 3.141592;
Console.WriteLine("i = " + i + ", x = " + x);
x = i; // 암시적 형변환
i = (int)x; // 캐스트가 필요함
Console.WriteLine("i = " + i + ", x = " + x);
}
}
}
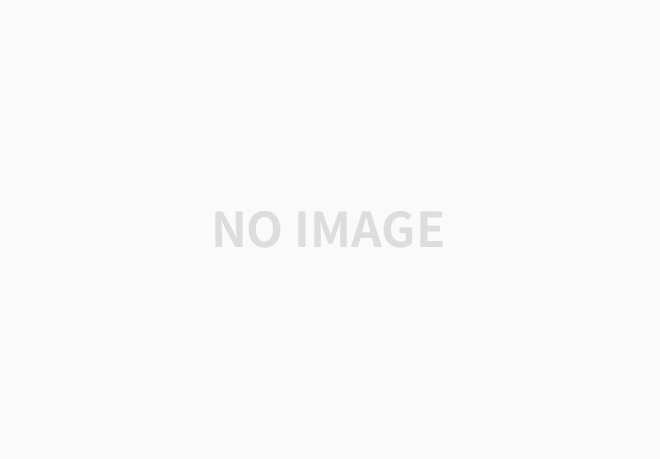
7. Console.WriteLine 메소드
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace A007_ConsoleWriteLine
{
class Program
{
static void Main(string[] args)
{
bool b = true;
char c = 'A';
decimal d = 1.234m; // m은 decimal형의 접미사
double e = 1.23456789;
float f = 1.23456789f; // f는 float형의 접미사
int i = 1234;
string s = "Hello";
Console.WriteLine("bool : " + b);
Console.WriteLine("char : " + c);
Console.WriteLine("decimal : " + d);
Console.WriteLine("double : " + e);
Console.WriteLine("float : " + f);
Console.WriteLine("int : " + i);
Console.WriteLine("string : " + s);
}
}
}
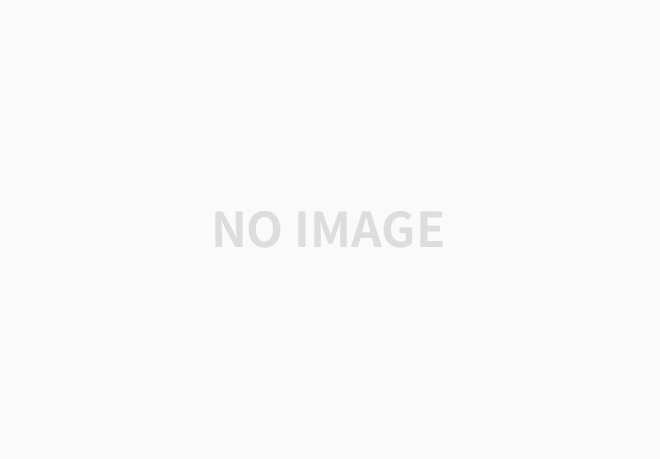
8. Console.WriteLine메소드로 여러개의 값을 출력
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace A008_ConsoleWriteLineMulti
{
class Program
{
static void Main(string[] args)
{
Console.WriteLine("10 이하의 소수 : {0}, {1}, {2}, {3}", 2, 3, 5, 7);
// String.Format을 사용하면 변수에 저장할 수 있음.
string primes;
primes = String.Format("10 이하의 소수 : {0}, {1}, {2}, {3}", 2, 3, 5, 7);
Console.WriteLine(primes);
}
}
}
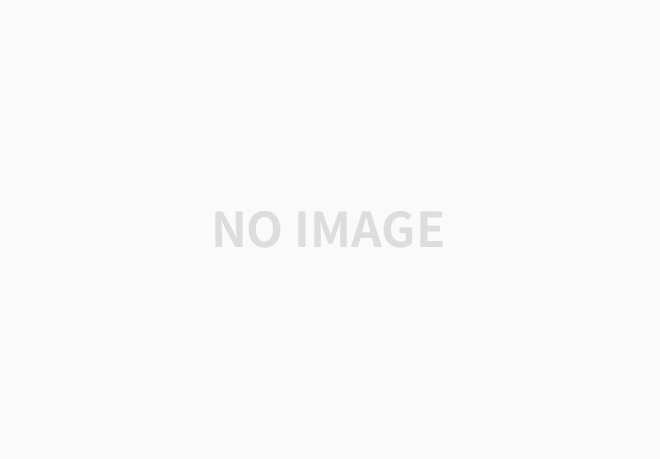
9.두 변수를 출력하는 방법
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace A009_VariablesAndWrite
{
class Program
{
static void Main(string[] args)
{
int v1 = 100;
double v2 = 1.234;
// Console.WriteLine(v1 + v2); 에러가 발생한다.
Console.WriteLine(v1.ToString() + ", " + v2.ToString());
Console.WriteLine("v1 = " + v1 + ", v2 = " + v2);
Console.WriteLine("v1 = {0}, v2 = {1}", v1, v2);
Console.WriteLine($"v1 = {v1}, v2 = {v2}");
}
}
}
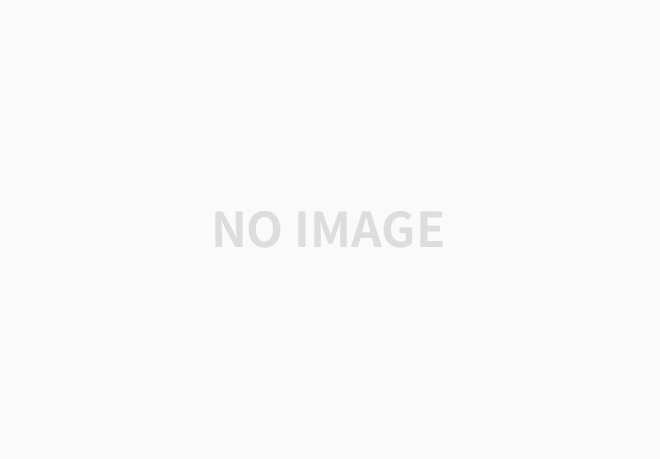
10. 형식지정자를 사용한 Console.WriteLine메소드
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace A010_ConsoleFormat
{
class Program
{
static void Main(string[] args)
{
Console.Clear();
Console.WriteLine("Standard Numeric Format Specifiers");
Console.WriteLine(
"(C) Currency : . . . . . {0:C}\n" +
"(D) Decimal :. . . . . . {0:D}\n" +
"(E) Scientific : . . . . {1:E}\n" +
"(F) Fiexed point : . . . {1:F}\n" +
"(G) General :. . . . . . {0:G}\n" +
"(N) Number : . . . . . . {0:N}\n" +
"(P) Percent :. . . . . . {1:P}\n" +
"(P) Round-trip : . . . . {1:R}\n" +
"(X) Hexadecimal :. . . . {0:X}\n",
-12345678, -1234.5678f);
}
}
}
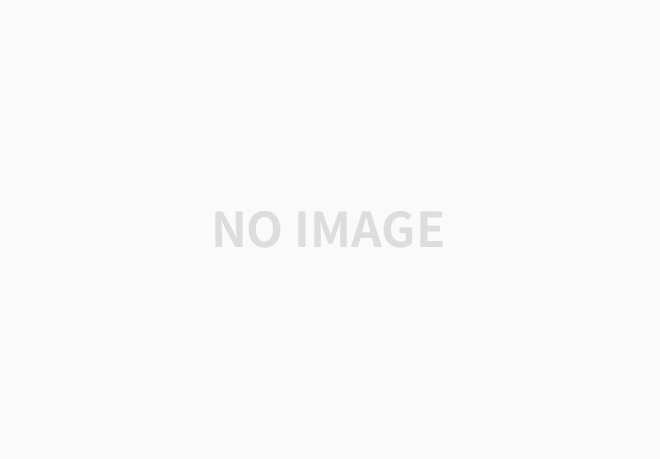
반응형
'C# > C#200제' 카테고리의 다른 글
[C#] 6일차 - 32. 열거형 enum (0) | 2021.01.21 |
---|---|
[C#] 5일차 (문자, 문자열 정리) (0) | 2021.01.20 |
[C#] 4일차 ( try~catch문, 각종 연산자 ) (0) | 2021.01.19 |
[C#] 3일차 ( Format과 연산자 처리) (0) | 2021.01.18 |
[C#] 1일차 (간단한 C#컴파일, 프로젝트만들기, 입출력) (0) | 2021.01.15 |
@반나무 :: 반나무_뿌리
3년차 WPF 개발자입니다.
포스팅이 좋았다면 "좋아요❤️" 또는 "구독👍🏻" 해주세요!